SoapClient
has the ability to enable tracing and give information about the request/response headers/body, which is useful, but I needed to store the outputs and also rewrite a particular request header during the course of debugging this. Enter: Charles Proxy. Continue reading Yearly Archives: 2013
PSR-What?
PSR
Let’s begin at the beginning. Once upon a time, at a conference, the lead developers from a selection of frameworks sat down in the same room (they are better at it nowadays, at the time I might not have believed it had I not been there) and agreed some standards for all their projects to use. The aim is to make PHP frameworks and libraries easier to combine for users, and so it was that php-fig: the PHP Framework Interop Group was born. This group of awesome individuals oversee the PHP Standards Recommendations (PSRs). Continue reading
Twitter Search API Using PHP and Guzzle
file_get_contents
in the general direction of the right URL. Continue reading Ttytter: Command Line Twitter Tweaks
I have customised a few settings which I find superhelpful, so I thought I’d share my config file and say a bit about some of the entries in there. The config for ttytter is held in a file called .ttytterrc
in my home directory. Mine looks like this: Continue reading
DC4D 6: Not-Programming for Programmers
Day Camp 4 Developers is a virtual conference, and it’s $40 (about 25 quid for UK people). If you can’t make it on the day, just get the video ticket and download the recorded sessions later. What I’m trying to say in this paragraph is that there are quite literally no excuses for missing out on this :)
Continue reading
Are Subqueries RESTful?
@lornajane sory for getting on your nervs with #rest,but are subquerys (like couchDB does) restfull to? is there a rule? like …/?type=bla
— Maximilian ‘Berghoff (@ElectricMaxxx) June 12, 2013
The blog seems like a good place, as I can put examples and all kinds other things here, and waffle at length (which is really why I like it!). Because when condensed to tweet form, the answer is really “it depends”.
The Problem(s)
REST is all about representations of resources. They might come in different formats, and they might appear at their own URI as well as in one or more collections, but essentially you just get a representation of a thing. This is great, apart from when it isn’t.
- What if you want a smaller result set with only a limited number of fields?
- What if you want related data? For every resource in a collection?
PHP Version Adoption
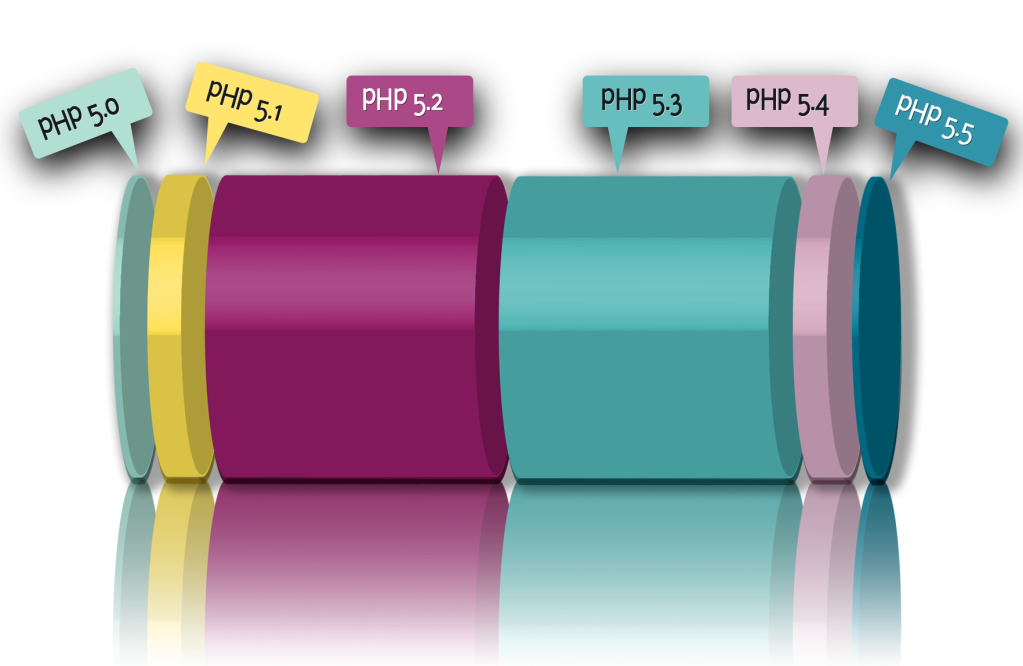
What’s alarming about this is that the left half of this graph represents unsupported versions of PHP. PHP 5.2 has been end of life since January 2011. This doesn’t mean that you can’t use it any more, but it does mean that in terms of security updates, you are out of luck. Some distributions will try to retro-fit some of the fixes but essentially your PHP applications seem a bit lacklustre because, well, you’re using technology from 2006. Continue reading
Simplest PHP Generator Example
Writing a Generator
The generators use the yield
keyword to feed values out as they are iterated over. In code, they really look a lot like a function (or method):
<?php
function getValues() {
// totally trivial example
yield "Apple";
yield "Ball";
yield "Cat";
}
Continue reading
Setting Multiple Headers in a PHP Stream Context
In fact, you’ve been able to pass this as an array since PHP 5.2.10, so to set multiple headers in the stream context, I just used this:
<?php $options = ["http" => [ "method" => "POST", "header" => ["Authorization: token " . $access_token, "Content-Type: application/json"], "content" => $data ]]; $context = stream_context_create($options);
The $access_token
had been set elsewhere (in fact I usually put credentials in a separate file and exclude it from source control in an effort not to spread my access credentials further than I mean to!), and $data
is already encoded as JSON. For completeness, you can make the POST request like this: